Administration
Configure cameras and manage parking spaces with extensive options. Monitor individual parking areas with multiple cameras to ensure efficiency. Create public visualization pages and set up real-time status updates via API interfaces.
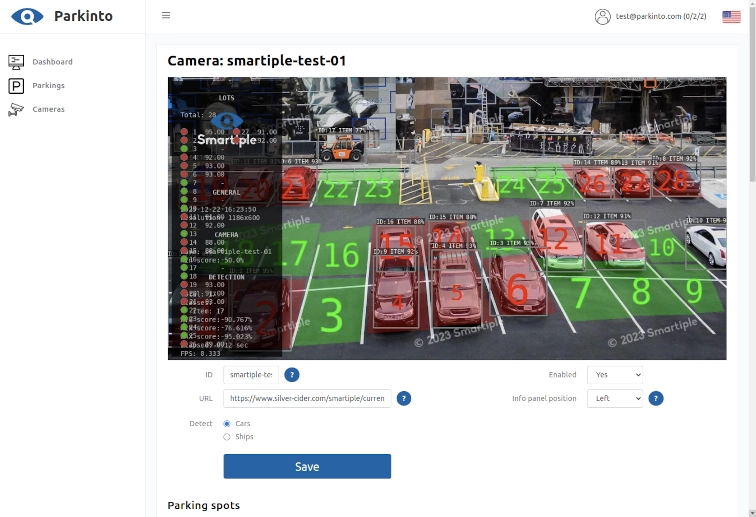
Configure Cameras and Manage Parking Spaces
We provide users with extensive options for managing their connected cameras. Users can configure the camera's access URL here allowing customization of the type of objects to be detected - whether cars or boats. Furthermore this section enables the definition and management of individual parking spaces monitored by the camera.
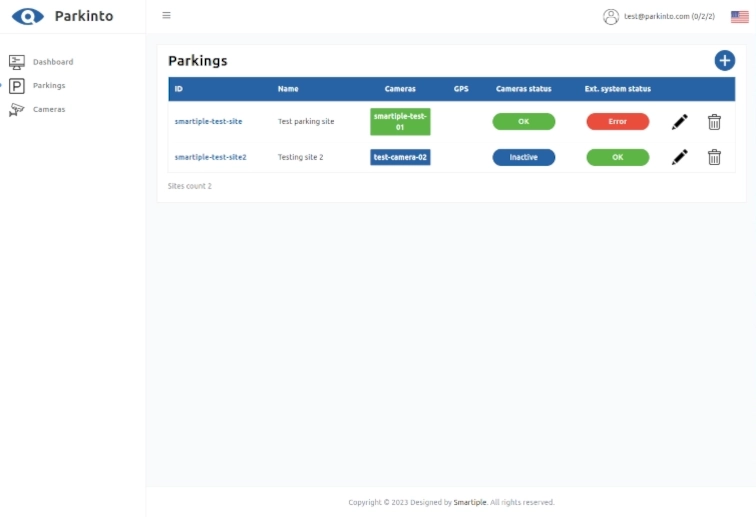
Multi-Camera Parking Area Management
This section is dedicated to managing individual parking areas. Each parking area can be monitored by multiple cameras simultaneously, providing users with the ability to ensure that all cameras are functioning properly and efficiently.
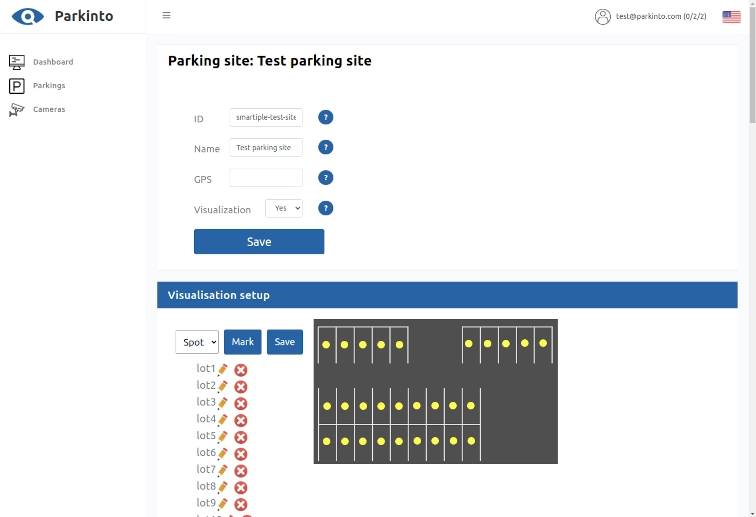
Real-Time Parking Visualization and Updates
Users have the capability to configure a so-called visualization page for each parking area. This is a publicly accessible address where the current status of the parking area is clearly displayed on a custom map. Additionally, users can configure external systems to receive real-time parking status updates via specified API interfaces.
API Integration
Communication with the Parkinto service is conducted via the HTTP/HTTPS protocol (REST API), where data messages are exchanged in the JSON format.
We offer two methods of communication with the Parkinto service. Customers may use either one or both simultaneously. In the first method (Request/Response), the customer can request the occupancy status of their site, with the response sent immediately. In the second method (Notification), the customer is notified by the Parkinto service. Notifications can be periodic (e.g., every 30 seconds) or triggered by changes in the occupancy status of the site.
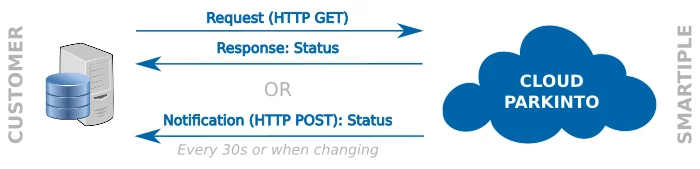
Python
import requests from requests.auth import HTTPBasicAuth # Define the API endpoint and parameters customer_name = "your_customer_name" site_name = "your_site_name" url = f"https://api.parkinto.com/v1/status/{customer_name}/{site_name}" # Define your login credentials username = "your_username" password = "your_password" # Make the GET request with basic authentication response = requests.get(url, auth=HTTPBasicAuth(username, password)) # Check the response status if response.status_code == 200: status_data = response.json().get('status_reply', {}) lots = status_data.get('lots', []) # Iterate over each lot and print its status for lot in lots: lot_name = lot.get('name') lot_state = lot.get('state') print(f"Lot Name: {lot_name}, State: {lot_state}") else: print("Failed to fetch status:", response.status_code)
JavaScript
const axios = require('axios'); // Define the API endpoint and parameters const customer_name = "your_customer_name"; const site_name = "your_site_name"; const url = `https://api.parkinto.com/v1/status/${customer_name}/${site_name}`; // Define your login credentials const username = "your_username"; const password = "your_password"; // Make the GET request with basic authentication axios.get(url, { auth: { username: username, password: password } }) .then(response => { const status_data = response.data.status_reply; const lots = status_data.lots; // Iterate over each lot and print its status lots.forEach(lot => { console.log(`Lot Name: ${lot.name}, State: ${lot.state}`); }); }) .catch(error => { console.error('Error fetching status:', error); });
PHP
<?php $customer_name = "your_customer_name"; $site_name = "your_site_name"; $url = "https://api.parkinto.com/v1/status/$customer_name/$site_name"; // Define your login credentials $username = "your_username"; $password = "your_password"; // Create a stream context for basic authentication $context = stream_context_create([ "http" => [ "header" => "Authorization: Basic " . base64_encode("$username:$password") ] ]); $response = file_get_contents($url, false, $context); if ($response !== FALSE) { $status_data = json_decode($response, true); $lots = $status_data['status_reply']['lots']; // Iterate over each lot and print its status foreach ($lots as $lot) { echo "Lot Name: " . $lot['name'] . ", State: " . $lot['state'] . "\n"; } } else { echo "Failed to fetch status\n"; } ?>
Bash
#!/bin/bash customer_name="your_customer_name" site_name="your_site_name" url="https://api.parkinto.com/v1/status/$customer_name/$site_name" # Define your login credentials username="your_username" password="your_password" # Make the GET request with basic authentication response=$(curl -s -u $username:$password $url) if [ $? -eq 0 ]; then lots=$(echo $response | jq -c '.status_reply.lots[]') echo "$lots" | while IFS= read -r lot; do lot_name=$(echo $lot | jq -r '.name') lot_state=$(echo $lot | jq -r '.state') echo "Lot Name: $lot_name, State: $lot_state" done else echo "Failed to fetch status" fi
Fetch Parking Occupancy Status
Learn how to use our API to get the current occupancy status of parking lots. Here are examples in Python, JavaScript, PHP, and Bash. Each example shows how to make a request and process the response. For detailed API documentation, visit our Swagger Documentation.
Python
from flask import Flask, request, jsonify app = Flask(__name__) @app.route('/webhook', methods=['POST']) def webhook(): data = request.json print('Received notification:') for lot in data['status_event']['lots']: print(f"Lot Name: {lot['name']}, State: {lot['state']}") return jsonify({'status': 'success'}) if __name__ == '__main__': app.run(port=5000)
JavaScript
const express = require('express'); const app = express(); app.use(express.json()); app.post('/webhook', (req, res) => { const data = req.body; console.log('Received notification:'); data.status_event.lots.forEach(lot => { console.log(`Lot Name: ${lot.name}, State: ${lot.state}`); }); res.status(200).send({ status: 'success' }); }); app.listen(5000, () => { console.log('Server is listening on port 5000'); });
PHP
<?php // Webhook endpoint if ($_SERVER['REQUEST_METHOD'] === 'POST') { $data = json_decode(file_get_contents('php://input'), true); error_log('Received notification:'); foreach ($data['status_event']['lots'] as $lot) { error_log('Lot Name: ' . $lot['name'] . ', State: ' . $lot['state']); } echo json_encode(['status' => 'success']); } else { http_response_code(405); echo 'Method Not Allowed'; } ?>
Bash
#!/bin/bash # Start a simple HTTP server to handle POST requests while true; do # Listen on port 5000 and respond to incoming POST requests response=$(nc -l -p 5000) lots=$(echo "$response" | jq -c '.status_event.lots[]') echo "Received notification:" echo "$lots" | while IFS= read -r lot; do lot_name=$(echo $lot | jq -r '.name') lot_state=$(echo $lot | jq -r '.state') echo "Lot Name: $lot_name, State: $lot_state" done echo "{\"status\":\"success\"}" done
Receive Parking Occupancy Notifications
Discover how our API can notify you of changes in parking occupancy. Here are examples in Python, JavaScript, PHP, and Bash for setting up notifications. For detailed API documentation, visit our Swagger Documentation.
Statistics
Unlock valuable insights with Parkinto's comprehensive parking data analysis. Easily access detailed reports and real-time data to optimize your parking management strategy.
Benefits for You:
- Smart Decisions: Use real data for better parking management.
- Identify Trends: See peak usage times.
- Efficient Resource Use: Allocate resources based on need.
- Improve Customer Experience: Enhance parking for your customers.
We offer two ways to get your statistics:
- View in Customer Portal: See your admin dashboard’s real-time data and detailed statistics. Easily monitor how each parking space is used. Examples of statistics you can see include:
- Average occupancy for the entire period
- Average parking occupancy for each day
- Average parking occupancy for each day of the week
- Histogram of parking usage by hour for each day of the week
- Maximum and minimum occupancy for the last week
- Receive CSV Reports via Email: Get detailed reports sent to your email in CSV format every month. Analyze parking usage with ease.
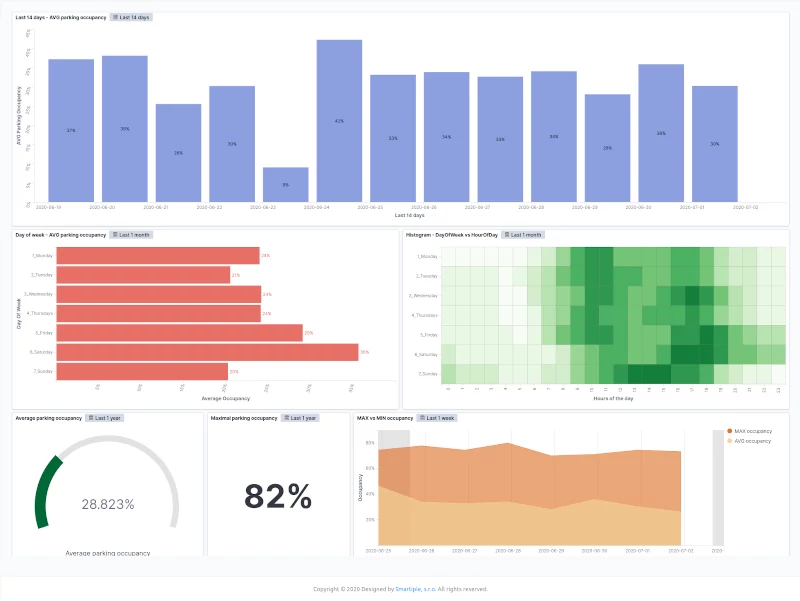
License Plate Detection
Get better parking control with our license plate recognition. Our system identifies cars accurately, works with your existing cameras, and includes access control without extra costs.
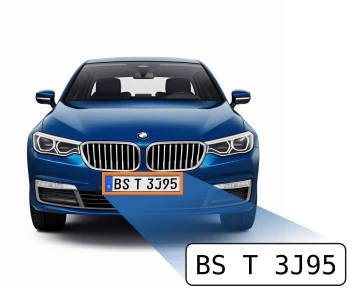
Visualization
Get instant access to parking occupancy and basic stats with our interactive map. Navigate easily to specific parking spots and visualize various types of parking spaces without logging in.
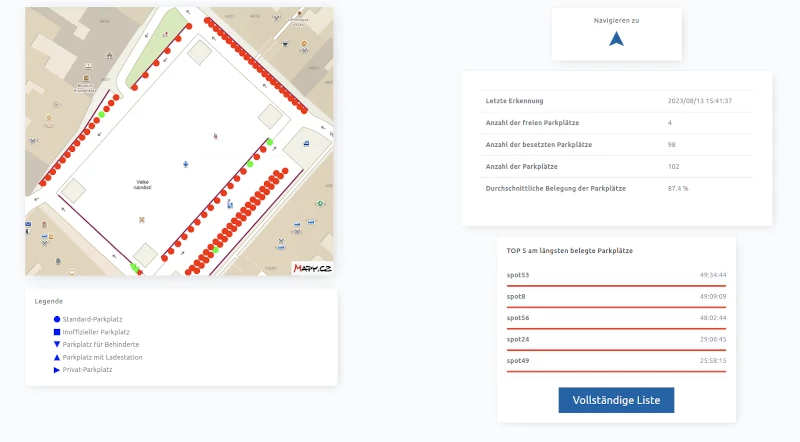
- Easy Access to Parking Occupancy and Stats:
Get a clear overview of parking occupancy on a map along with basic statistical data. - No Login Required:
Access this feature directly via a specific URL. - Responsive Design:
Optimized for mobile devices, allowing easy navigation to specific parking spots. - Visualize Different Parking Types:
Supports various parking spots: Standard, Unofficial, Reserved for Disabled, Recharger, and Private. - Multi-language Support:
Available in English, German, Spanish, Danish, Norwegian, and Czech.
Mobile App
Our Parkinto mobile app provides users with an easy way to find and navigate to available parking spaces in the city. It integrates real-time data and navigation features to ensure a stress-free parking experience.
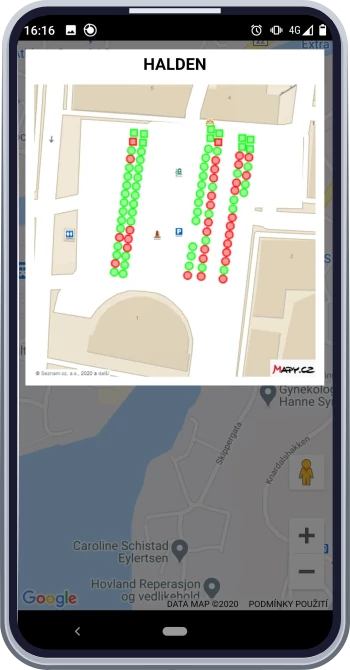
Need Help?
Didn't find what you were looking for? Need more detailed information? Contact us, and we'll be happy to assist you.